The term ‘Pseudo Random’ itself exaplins most about the concept. Pseudo Random Numbers is a sequence that seems statistically random, but is generated by deterministic algorithm based on a starting value called seed.
Computers, or programs, are entirely predictable by design. That is anything it generates randomnly are not truly random. Because, when an algorithm functions correctly, nothing it does is random. So, to generate numbers that are unpredictable, programs use mathematical algorithms to produce numbers that are ‘random enough’.
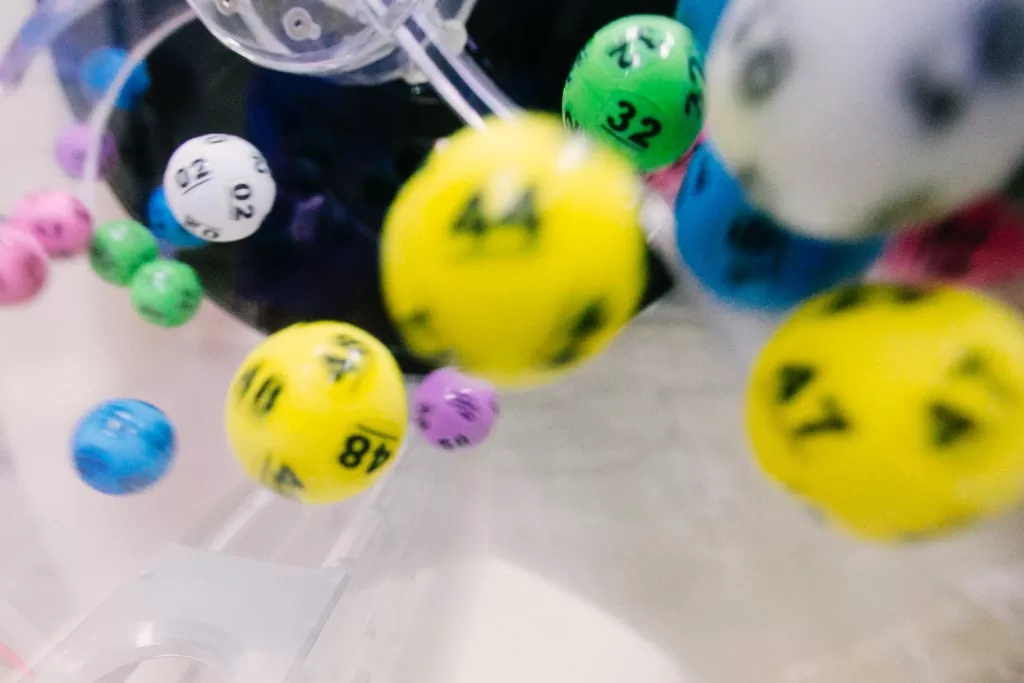
For example, if a computer program generates random numbers using seed value of 10, it will produce a predictable sequence of numbers. Each time the program is run with the same seed value, it will produce the same sequence of numbers.
What are Pseudo Random Number Generators (PRNGs)
A pseudo random number generator (PRNG) is an algorithm that generates a sequence of numbers that appear to be random, but are actually deterministic. It uses an initial value, called a seed, to generate a sequence of numbers that are statistically indistinguishable from true random numbers. The same seed will always produce the same sequence of numbers, making the sequence predictable.
PRNGs are widely used in various fields, such as cryptography, simulations, gaming, and data analysis, where random numbers are needed. The quality of a PRNG is evaluated based on its randomness, period, and distribution of numbers generated. Common PRNGs include linear congruential generators, Mersenne Twister, and Xorshift.
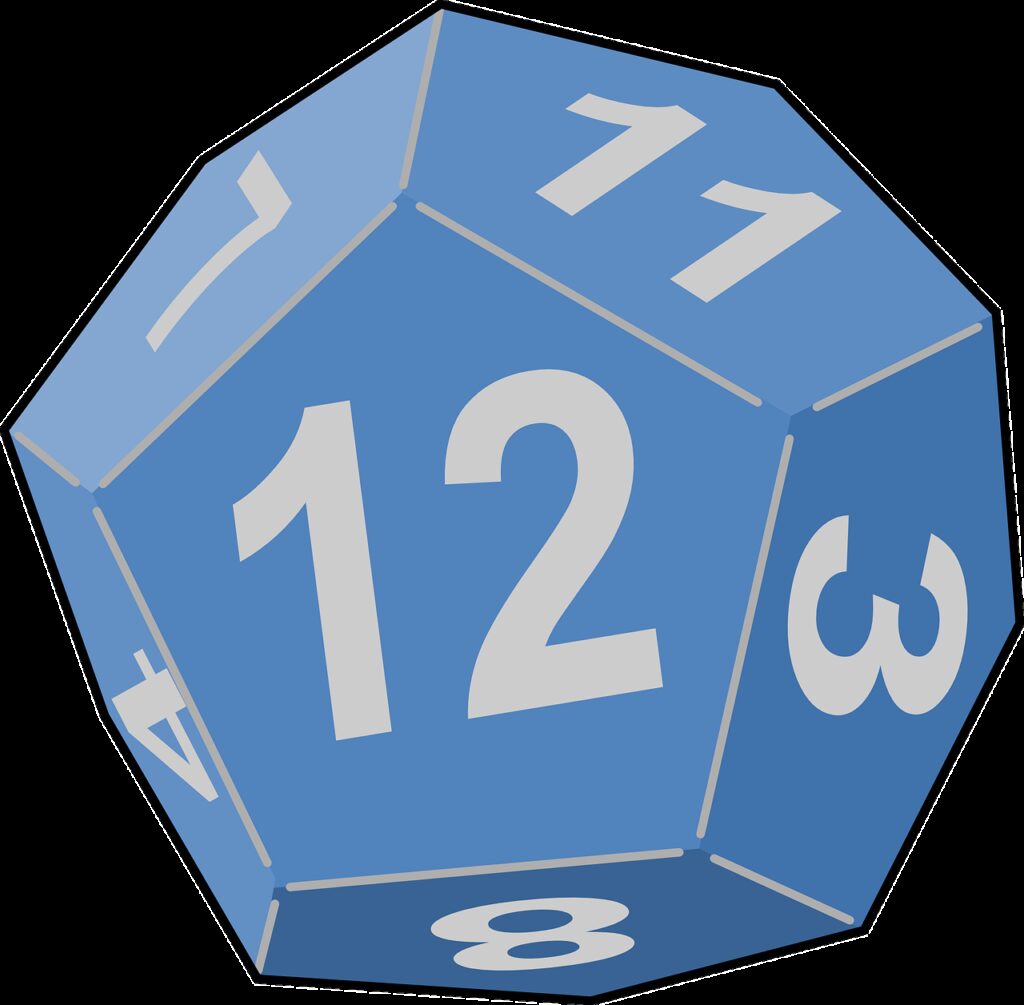
How Pseudo Random Numbers are Generated in Python
In Python, the random module provides functions to generate pseudo random numbers. Here are a few examples:
- Random Integer: To generate a random integer between a given range, use the randint function:
import random
random.randint(1, 10)
- Random Float: To generate a random float between 0 and 1, use the random function:
import random
random.random()
- Random Element: To select a random element from a list, use the choice function:
import random
random.choice([1, 2, 3, 4, 5])
- Random Sampling: To generate a random sample from a population, use the sample function:
import random random.sample([1, 2, 3, 4, 5], 3)
How Pseudo Random Numbers are used in Data Analytics

Following use cases show how pseudo random numbers are widely used in data analytics to improve the accuracy, efficiency and robustness of analytical models and results.
1. Sampling
In large datasets, pseudo random numbers can be used to sample a representative subset of data for analysis, reducing computational cost and improving efficiency.
2. Monte Carlo Simulations
Pseudo random numbers are used to perform Monte Carlo simulations for forecasting and risk analysis.
3. Data Shuffling
Pseudo random numbers can be used to shuffle data for cross-validation, or to create randomized control groups in experiments.
4. Data Augmentation
Pseudo random numbers can be used to augment data by adding random noise or missing data patterns, to evaluate the robustness of analytical models.
5. Randomized Algorithms
Many algorithms in data analytics, such as randomized linear algebra, use pseudo random numbers to find approximate solutions to large scale problems.
How Pseudo Random Numbers are used in ML, DL and AI Models
Pseudo random numbers play an important role in machine learning, deep learning and artificial intelligence models. Following are the most important use cases:
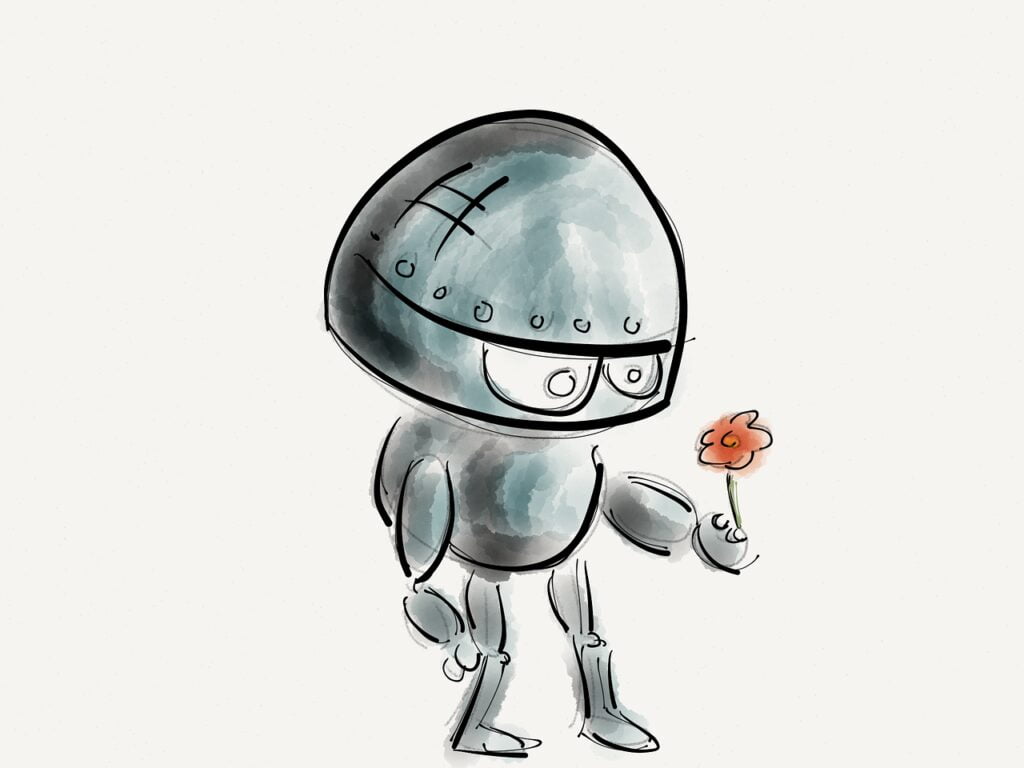
1. Data Splitting
Pseudo random numbers are used to randomly split data into training, validation, and testing sets.
2. Data Augmentation
In computer vision and image classification, pseudo random numbers are used to augment data by applying random transformations, such as rotation, scaling, and flipping, to the original data.
3. Model Initialization
Random initialization of weights is a common practice in deep learning or neural network models to avoid getting stuck in local optima and improve convergence of the model.
4. Exploration and Exploitation
In reinforcement learning, pseudo random numbers are used to balance exploration and exploitation, where the model explores new actions to learn and exploits learned actions for maximum rewards.
5. Stochastic Processes
Many machine learning algorithms, such as Monte Carlo simulations and Markov Chain Monte Carlo (MCMC) methods, use pseudo random numbers in order to model random processes. Deep learning algorithms, such as Generative Adversarial Networks (GANs) and Reinforcement Learning, use pseudo random numbers for modeling random processes.
6. Regularization
Dropout, a common regularization technique used in deep learning, uses pseudo random numbers to randomly drop out some neurons during training, improving model generalization.
Applications of Pseudo Random Numbers in Real Life
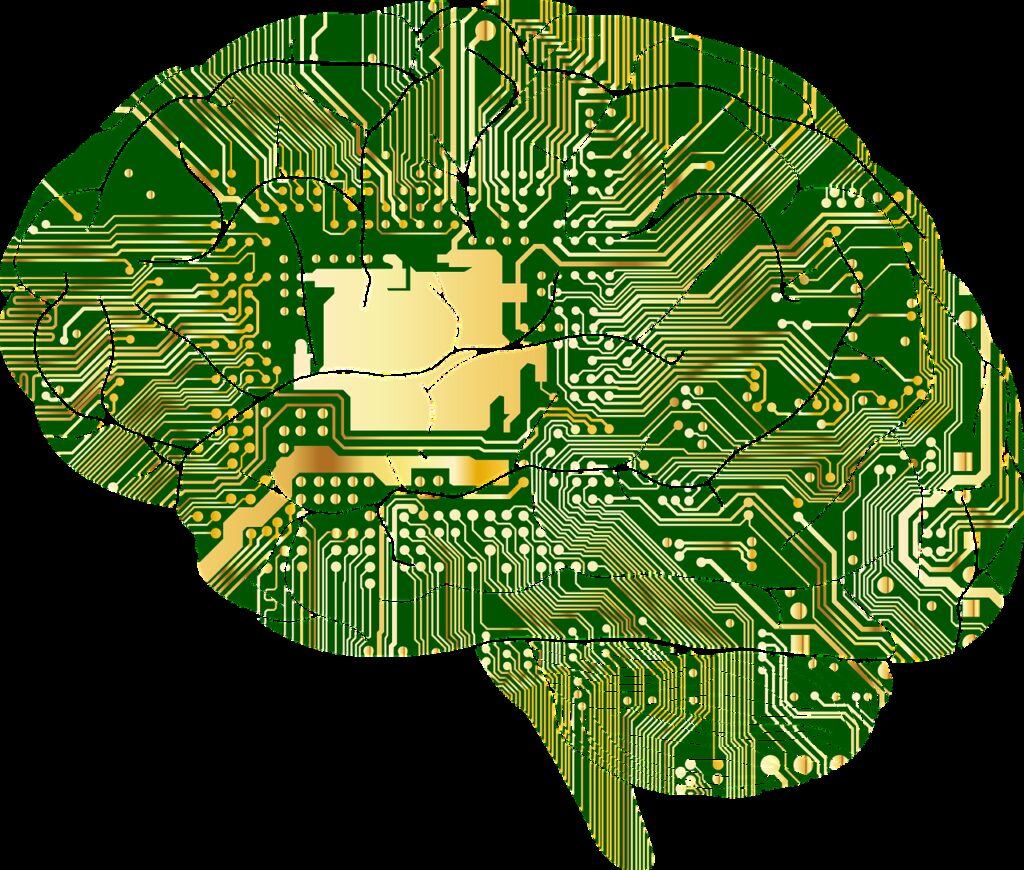
Following examples show how pseudo random numbers are widely used in real life to solve a variety of problems, from securing sensitive information to modeling complex systems.
1. Cryptography
Pseudo random numbers are used to generate secure encryption keys in cryptography, ensuring the privacy and security of sensitive information.
2. Simulation
Pseudo random numbers are used to model and simulate complex systems in various fields, such as finance, engineering, and climate science.
3. Gaming
Pseudo random numbers are used to generate random events and outcomes in computer games, such as rolling dice, shuffling cards, and spinning wheels.
4. Sampling
In market research and surveys, pseudo random numbers are used to select a representative sample of people for interviews and data collection.
5. Pseudo Random Bit Generation
Pseudo random bit generators are used in various fields, such as random number generation for scientific simulations, randomization in controlled clinical trials, etc.
6. Physical Random Number Generation
Pseudo random numbers generated from physical phenomena, such as atmospheric noise or radioactive decay, are used in cryptography and secure communication.
More to Read:
- “Pseudorandomness”. Foundations and Trends in Theoretical Computer Science, by Vadhan S.P. (2012)
- Randomness Requirements for Security, by D. Eastlake, 3rd; J. Schiller; S. Crocker (2005)
Please, don’t forget to subscribe newsletter for updates on new articles and upcoming exciting sections.